The Java do-while loop is used to iterate a set of statements until the given condition is satisfied. If the number of iteration is not fixed and you MUST have to execute the loop at least once, then use a do while loop.
What is Do while loop in Java?
Do-while loop is similar to while loop, but it possesses one dissimilarity: In while loop, the condition is evaluated before the execution of loop’s body but in do-while loop condition is evaluated after the execution of loop’s body.
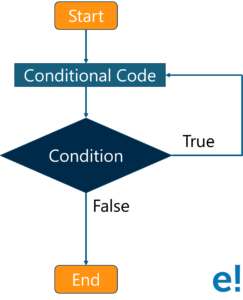
Let me explain the above-shown diagram:
First of all, it will execute a set of statements that are mentioned in your ‘do’ block.
After that, it will come to ‘while’ part where it checks the condition.
If the condition is true, it goes back and executes the statements.
If the condition is false, it directly exits the loop.
public class DoWhileExample
{
public static void main(String[] args)
{
int i=1;
do
{
System.out.println(i);
i++;
}
while(i<10);
}
}

What is for loop?
Programmers usually use loops to execute a set of statements. For loop is used when they need to iterate a part of the programs multiple times. It is particularly used in cases where the number of iterations is fixed
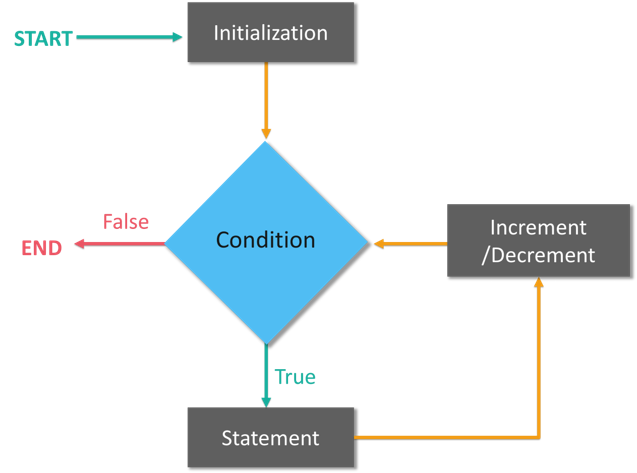
Java nested for loop
If you have a for loop inside a for loop, you have encountered a Java nested for loop. The inner loop executes completely when the outer loop executes.
class Example{
public static void main(String args[]){
int val = 1;
for(int count = 1;count<2;++count)
{
for(int i=1;i<=3;i++)
{
System.out.print(val+” “);
}
val++;
//System.out.println();
}
}
}

reference: https://www.edureka.co/blog/java-for-loop